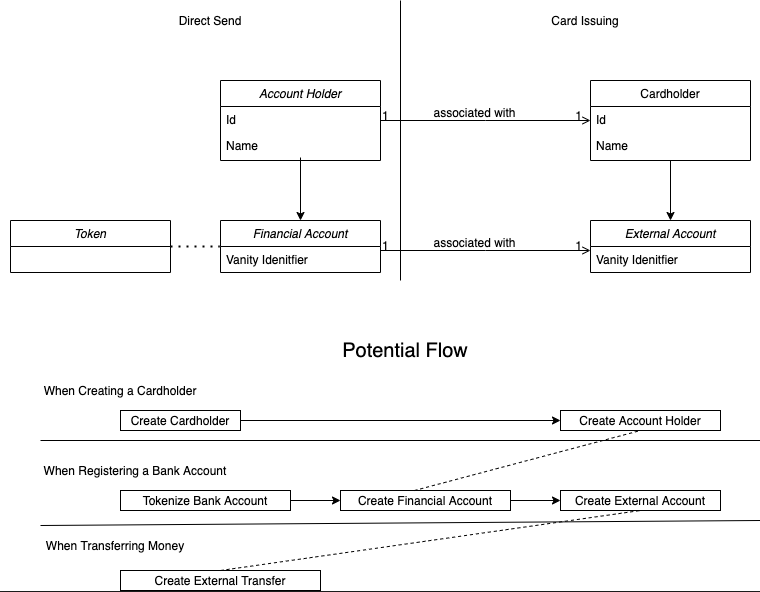
Step 1: Tokenize Bank Account
POST {{domain}}/api/v1/tokens
{
"bank_account": {
"account_number": "12341234",
"account_holder_name": "John Smith",
"transit_number": "12345",
"institution_number": "001",
"currency": "CAD",
"country": "CAN"
}
}
{
"data": {
"account_holder_name": "John Smith",
"account_number": "12341234",
"country": "CAN",
"currency": "CAD",
"institution_number": "001",
"last_four_digits": "1234",
"risk_level": "medium",
"routing_number": "000112345",
"token": "batk_Qga-ueYOlW7KAhkgZ5DtF4406812DFC3C1234",
"transit_number": "12345",
"type": "bank_account"
}
}
Step 2: Create Account Holder
POST {{domain}}/api/v1/account_holders
{
"type": "individual",
"email": "[email protected]",
"first_name": "John",
"last_name": "Smith"
}
{
"data": {
"email": "[email protected]",
"id": 5,
"type": "individual"
}
}
Step 3: Create Financial Account
POST {{domain}}/api/v1/account_holders/:id/financial_accounts
{
"token": "batk_Qga-ueYOlW7KAhkgZ5DtF4406812DFC3C1234"
}
{
"data": {
"account_holder_id": 3,
"account_holder_name": "John Smith",
"account_number": "12341234",
"country": "CAN",
"currency": "CAD",
"id": "eft_3_batk_Qga-ueYOlW7KAhkgZ5DtF4406812DFC3C1234",
"institution_number": "001",
"last_four_digits": "1234",
"network_type": "eft",
"risk_level": "medium",
"routing_number": "000112345",
"status": "active",
"transit_number": "12345",
"type": "bank_account"
}
}
Card Issuing
Step 1: Create an External Account
POST '{{domain}}/api/v1/card_issuing/cardholders/:id/external_accounts' \
{
"data": {
"id": 28,
"name": "My Scocia Account",
"type": "personal_account",
"vanity_identifier": "eft_3_batk_Qga-ueYOlW7KAhkgZ5DtF4406812DFC3C1234"
}
}
{
"data": {
"cardholder_id": 418,
"created_at": "2021-02-09T02:21:08Z",
"id": 28,
"name": "My Scocia Account",
"type": "person_to_person",
"updated_at": "2021-02-09T02:21:08Z"
}
}
Step 2: Create Transaction
POST '{{domain}}/api/v1/card_issuing/external_transfers'
{
"account_id": 20,
"external_account_id": 1,
"amount": 1000,
"transfer_type": "direct_send_transaction",
"external_tag": "DEMO",
"message": "For Me",
"descriptor": "descriptor", // Optional, EFT only. Description that will appear on the EFT recipt. Has a default set up by operations.
"originator_id": 1, // Optional, EFT only. Originator short and long name that will appear on the EFT recipt. Has a default set up by operations.
}
{
"data": {
"id": 22,
"company_id": "1",
"program_id": 44,
"cardholder_id": 418,
"account_id": 20,
"external_account_id": 1,
"external_tag": "DEMO",
"transfer_type": "direct_send_transaction",
"direct_send_reference": "dasads4554af55".
"amount": 2000,
"message": "Test",
"direct_send_status": "approved",
"created_at": "2020-09-11T20:03:52Z",
"updated_at": "2020-09-11T20:03:54Z"
}
}
Originator Names
Counterparty Financial Institution | Counterparty Financial Institution Bank Statement EFT Description Mapping |
BMO | [Originator Short Name] + [CPA Code Abbreviation] |
CIBC | [Transaction Descriptor] + [Originator Long Name] |
Desjardins | [Transaction Flow] + [Originator Long Name] |
RBC | [CPA Code Full Description] + [Originator Short Name] |
Scotiabank | [CPA Code Full Description] + [Originator Long Name] |
TD Canada Trust | [Originator Short Name] + [CPA Code Abbreviation] |
Field | Field Description |
Originator Short Name (15 characters) | Your company name in short form. Berkeley will capture this when setting up your production service account |
Originator Long Name (30 characters) | Your company name. Berkeley will capture this when setting up your production service account |
CPA Code Full Description | CPA Code full description as referenced in Standard 007 (“Full” column) |
CPA Code Abbreviation | CPA Code full description as referenced in Standard 007 (“Abbreviations” column) |
Transaction Flow | A description indicating whether the transaction is a CREDIT or a DEBIT |
Transaction Descriptor (15 characters) | This field is used to provide additional information to identify the transaction to the payee/payor e.g. pay period, loan number, insurance policy number, etc. You can optional provide this information on a transactional basis through the transactionDescriptor field. Refer to: 6.4.1Creating an EFTCreating an EFT |
Related pages:
API Flows
Card Issuing API Quickstart Guide
Codes, Statuses and Errors